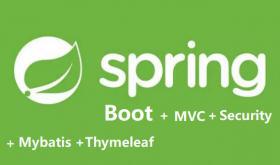
本教程架构当前较流行的 Java 技术:
Spring Boot、MVC 、Security + Mybatis + Mysql + Thymeleaf
Spring Security 功能强大,详细去官网了解。
创建以下包:
- com.example.demo.security
追加 Security 类:
package com.example.demo.security; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import com.example.demo.service.UserDetailsServiceImpl; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Bean UserDetailsService fetchUserDetailsService() { return new UserDetailsServiceImpl(); } @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.userDetailsService(fetchUserDetailsService()).passwordEncoder(new BCryptPasswordEncoder()); } @Override protected void configure(HttpSecurity http) throws Exception { http.authorizeRequests() .antMatchers("/admin/**").hasAuthority("Administrator") .anyRequest().authenticated() .and() .formLogin() .permitAll() .and() .logout() .permitAll(); } }
追加 Service 类:
package com.example.demo.service; import java.util.ArrayList; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.core.authority.SimpleGrantedAuthority; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.core.userdetails.UsernameNotFoundException; import com.example.demo.entity.User; import com.example.demo.mapper.UserMapper; public class UserDetailsServiceImpl implements UserDetailsService { @Autowired private UserMapper userMapper; @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { User user = userMapper.fetchUserByUsername(username); if (user == null) { throw new UsernameNotFoundException("Invalid User!"); } List<SimpleGrantedAuthority> authorities = new ArrayList<>(); authorities.add(new SimpleGrantedAuthority("Administrator")); return new org.springframework.security.core.userdetails.User( user.getUsername(), user.getPassword(), authorities); } }